목차
문제 설명
다음 그림과 같이 지뢰가 있는 지역과 지뢰에 인접한 위, 아래, 좌, 우 대각선 칸을 모두 위험지역으로 분류합니다.
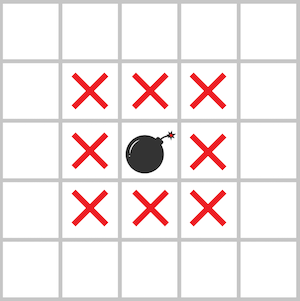
지뢰는 2차원 배열 board에 1로 표시되어 있고 board에는 지뢰가 매설 된 지역 1과, 지뢰가 없는 지역 0만 존재합니다.
지뢰가 매설된 지역의 지도 board가 매개변수로 주어질 때, 안전한 지역의 칸 수를 return하도록 solution 함수를 완성해주세요.
제한사항
- board는 n * n 배열입니다.
- 1 ≤ n ≤ 100
- 지뢰는 1로 표시되어 있습니다.
- board에는 지뢰가 있는 지역 1과 지뢰가 없는 지역 0만 존재합니다.
board | result |
---|---|
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 1, 0, 0], [0, 0, 0, 0, 0]] | 16 |
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 1, 1, 0], [0, 0, 0, 0, 0]] | 13 |
[[1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1]] | 0 |
class Solution {
public int solution(int[][] board) {
int len = board.length;
int[][] temp_board = board;
for(int i = 0; i < len; i++){
for(int j = 0; j < len; j++){
if(board[i][j] == 1){
if(0 <= i - 1 && 0 <= j - 1){
if((temp_board[i-1][j-1] != 1)){
temp_board[i-1][j-1] = 2;
}
}
if(0 <= i - 1 && 0 <= j){
if((temp_board[i-1][j] != 1)){
temp_board[i-1][j] = 2;
}
}
if(0 <= i - 1 && (0 <= j + 1 && j + 1 < len)){
if((temp_board[i-1][j+1] != 1)){
temp_board[i-1][j+1] = 2;
}
}
if(0 <= i && 0 <= j - 1){
if((temp_board[i][j-1] != 1)){
temp_board[i][j-1] = 2;
}
}
if(0 <= i && (0 <= j + 1 && j + 1 < len)){
if((temp_board[i][j+1] != 1)){
temp_board[i][j+1] = 2;
}
}
if((0 <= i + 1 && i + 1 < len) && 0 <= j - 1){
if((temp_board[i+1][j-1] != 1)){
temp_board[i+1][j-1] = 2;
}
}
if((0 <= i + 1 && i + 1 < len) && 0 <= j){
if((temp_board[i+1][j] != 1)){
temp_board[i+1][j] = 2;
}
}
if((0 <= i + 1 && i + 1 < len) && (0 <= j + 1 && j + 1 < len)){
if((temp_board[i+1][j+1] != 1)){
temp_board[i+1][j+1] = 2;
}
}
}
}
}
int cnt = 0;
for(int[] x : temp_board){
for(int y: x){
if(y == 0){
cnt++;
}
}
}
return cnt;
}
}
2중 for문 안의 2번째 if문안의 if문이 총 8개 있는데 이는 칠해야할 안전지대를 말한다.
1 번째 if문 | 2 번째 if문 | 3 번째 if문 |
4 번째 if문 | 지뢰 | 5 번째 if문 |
6 번째 if문 | 7 번째 if문 | 8 번째 if문 |
class Solution {
public int solution(int[][] board) {
int len = board.length;
int[][] temp_board = new int[len][len];
// 지뢰 및 주변 지역 표시
for (int i = 0; i < len; i++) {
for (int j = 0; j < len; j++) {
if (board[i][j] == 1) {
// 지뢰 위치 및 주변 8방향 표시
for (int di = -1; di <= 1; di++) {
for (int dj = -1; dj <= 1; dj++) {
int ni = i + di;
int nj = j + dj;
if (ni >= 0 && ni < len && nj >= 0 && nj < len && board[ni][nj] != 1) {
temp_board[ni][nj] = 2; // 위험 지역 표시
}
}
}
}
}
}
// 안전한 지역 카운트
int cnt = 0;
for (int i = 0; i < len; i++) {
for (int j = 0; j < len; j++) {
if (board[i][j] == 0 && temp_board[i][j] == 0) {
cnt++;
}
}
}
return cnt;
}
}
if문의 개수가 너무 많고 해석하기 어려울 것 같아 최적화를 시도해봤다.
728x90
'JAVA > 프로그래머스 코딩테스트 입문' 카테고리의 다른 글
[프로그래머스 , JAVA, LV.0] 평행 (0) | 2024.12.17 |
---|---|
[프로그래머스, JAVA, LV.0] 겹치는 선분의 길이 (0) | 2024.12.17 |
[프로그래머스 LV.0] 연속된 수의 합 (2) | 2024.12.15 |
[프로그래머스, LV.0] 다음에 올 숫자 (0) | 2024.12.15 |
[프로그래머스, LV.0] OX퀴즈 (0) | 2024.12.15 |